This project version modifies the previous one in the following way: Instead of charting the sensor data on the screen, the application records the sensor data in a Log.
Logs are special data tables that can only be appended to. There may only be one log table per device. Log table structure needs not to be defined in advance -- your application may add log columns on the fly. There is no way for the application to clear the log. This can only be done by the user through the web interface.
Logging is enabled on the Log page of the Features tab. Records are added using the Add to Log block. Click on this block in the current application to examine its properties:
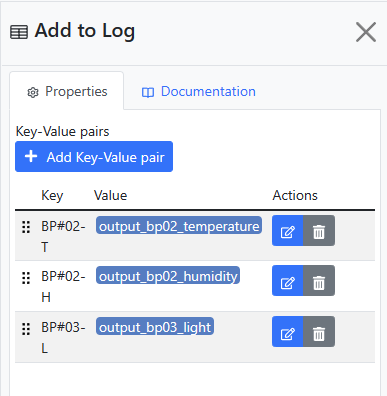
As you can see, log records consist of key-value pairs. The key is the name of the field (column) the data will go into. The value is the data itself. Log fields are stored in the string format, so there is no division into data types here.
If an invocation of the Add to Log block defines a key (column) that doesn't yet exist, this column is added before appending a new record. Add to Log blocks do not have to fill out every existing field -- supplying partial data leaves the remaining fields blank.
Calling the Add to Log block with key1-value and then calling this block again with key2-value is different than calling the block once with both key1 and key2 present. The first sequence will result in adding two records. Both records will have one of the fields filled in and one of the fields empty. Only one record will be added in the second case, and it will have data in both fields.
This application supplies all the key-value pairs every time. Here is how the log looks in the web interface:
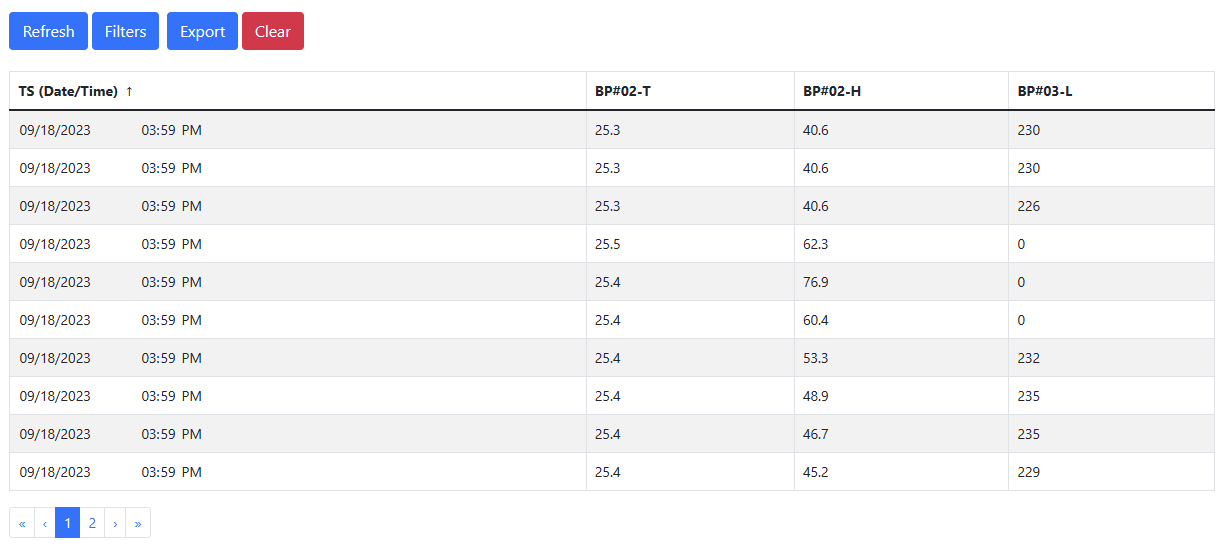